Image filters
Image filters can be plugged in to enhance images during album generation, adding watermarking, logos etc.
Image filters can be applied to closeup images, thumbnail images or both. Several filters can be chained to produce the desired effect. Imagine first applying red-eye reduction, then sharpening images and finally adding your logo to a corner. Well, all these filters are not written at the moment, but I expect them to show up: An open API and source code example are provided so developers easily can add new filters. Currently there is no graphical user interface for image filters, but with some fairly simple settings in the "User defined variables" section of the Advanced page, they can be controlled.
Quick example
Learning by example is powerful, so let's start by applying a grayscale filter to each thumbnail and add a copyright notice to the corner of each closeup image. (For a formal description of available filter features, see below.)
Start JAlbum and prepare a project for album creation. Now go to the Advanced page of JAlbum. There you will find a section called "User defined variables". We will use this section to set the properties of our filters. Enter exactly as this example shows. Pay attention to capitalization, ("GrayscaleFilter" is different to Grayscalefilter"). When done, remember to press ENTER to store the new settings.
Setting filter properties on the Advanced page
Now go to the Main page and press "Rebuild all" to have the album generated and filters applied. (Pressing "Rebuild all" is important as pressing "Make album" does not process images if they already exist from a previous album generation.) The resulting thumbnails and closeup images should now look something like this:
Thumbnail and closeup images processed through grayscale and text filters
The filters are applied in the order they are numbered (filter1, filter2 etc). The filter settings gets saved with the album project so don't forget to save your project when you are happy with your settings.
The filters
Below is a list of the initially provided filters and their properties (features). New filters can be installed by putting their Java class files inside the plugins directory of JAlbum. All filters require the class property to be specified as filter identifier. All filters accept the thumbnails and closeups property that controls if the filter is to be applied to thumbnails only, closeups only or both. Default is both.
Grayscale filter
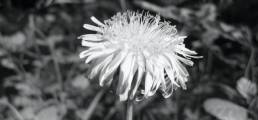
Converts images to grayscale.
Property |
Values |
Comment |
class |
GrayscaleFilter |
Identifying filter name (required) |
thumbnails |
true|false |
Apply to thumbnails? |
closeups |
true|false |
Apply to closeups? |
Example: class=GrayscaleFilter
Applies the grayscale filter to both thumbnails and closeups.
Logotype filter
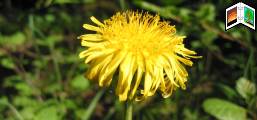
Adds image/logotype on top of album images.
Property |
Values |
Comment |
class |
LogoFilter |
Identifying filter name (required) |
thumbnails |
true|false |
Apply to thumbnails? |
closeups |
true|false |
Apply to closeups? |
src |
URL to jpeg or gif image |
Any valid URL will do |
align |
left|center|right |
Horizontal alignment of logotype (left) |
valign |
top|center|bottom |
Vertical alignment of logotype. (top) |
margin |
Margin in pixels |
Distance from edge of logotype to edge of image. (0) |
offset |
Horizontal offset in pixels |
Fine tuning of placement. (0) |
voffset |
Vertical offset in pixels |
Fine tuning of placement. (0) |
Example: class=LogoFilter src="http://www.datadosen.se/jalbum/images/bigicon.gif" closeups align=right
Add the JAlbum logo from the JAlbum web site to the right corner of closeups.
Text filter
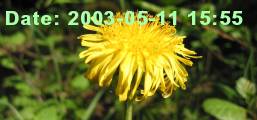
Adds texts to album images.
Property |
Values |
Comment |
class |
TextFilter |
Identifying filter name (required) |
thumbnails |
true|false |
Apply to thumbnails? |
closeups |
true|false |
Apply to closeups? |
text |
Text to display |
Allows JAlbum $variables like $originalDate and $comment to be embedded for dynamic content |
color |
HTML color definition |
Understands common Netscape and Explorer color names like "pink", #rrggbb and r,g,b,a format |
face |
Name of font |
Valid font names from your system like "Helvetica", "Verdana", "Courier New" etc |
style |
plain|bold|italic|bolditalic |
Font style (bold) |
size |
Font size in pixels |
(16) |
antialias |
true|false |
Antialiasing gives smoother edges. (true) |
align |
left|center|right |
Horizontal alignment of text (left) |
valign |
top|center|bottom |
Vertical alignment of text. (top) |
margin |
Margin in pixels |
Distance from edge of text to edge of image. (5) |
offset |
Horizontal offset in pixels |
Fine tuning of placement. (0) |
voffset |
Vertical offset in pixels |
Fine tuning of placement. (0) |
Example: class=TextFilter text="Date: $fileDate" size=20 color=#aaffaa closeups
Add the file date of the image on top of the image in a light green color
Shadow text filter
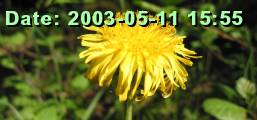
Like text filter but also adds a drop shadow.
Extended properties (see TextFilter for the others):
Property |
Values |
Comment |
class |
ShadowTextFilter |
Identifying filter name (required) |
shadowColor |
HTML color definition |
Color of shadow. Understands common Netscape and Explorer color names like "pink", #rrggbb and r,g,b,a format |
shadowDistance |
Distance in pixels |
(2) |
Example: class=ShadowTextFilter text="Date: $fileDate" size=20 color=#aaffaa closeups
Add the file date of the image on top of the image in a light green color with a black shadow
Watermark filter
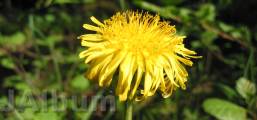
Like text filter but with a watermark effect.
Extended properties (see TextFilter for the others):
Property |
Values |
Comment |
class |
WatermarkFilter |
Identifying filter name (required) |
strengthPercent |
Strength in percent |
Controls watermark visibility. (20) |
Example: class=WatermarkFilter text="JAlbum" size=30 closeups valign=bottom margin=0
Add a "JAlbum" watermark to the lower left edge
Adding filters to skins
Skins can be configured to automatically use certain filter settings. Simply save a project containing suitable filter settings in the "User defined variables" section, then rename the project file to hints.jap and put it in the skin folder. JAlbum will now load filter settings from this file when such a skin is selected. Remember to also strip the hints file from other settings that you don't want to be applied.
Developing filters
This section is for Java programmers who wish to extend JAlbum with new image filters.
JAlbum image filters are java classes added to the plugins directory that implement the small se.datadosen.jalbum.JAFilter interface. A JAlbum filter should also adhere to the JavaBean specification, meaning:
- Provide a no-args constructor
- Getters and setter methods should follow the JavaBean naming convention.
- The filter should be serializable
A se.datadosen.jalbum.JAFilter interface must provide getter methods for name and description pretty much like an Applet. The third method is the filter method that takes a BufferedImage and a java.util.Map as arguments and returns a modified BufferedImage . A filter is allowed to directly manipulate the passed BufferedImage . The Map object contains JAlbum $variables that may be useful for the filter implementer.
The preferred way to pass parameters to a filter is to implement setter methods for them. By using a smart BeanShell script in the init.hsh file in the JAlbum program directory, JAlbum is capable of passing attributes from the user defined variables section of the Advanced tab page to the corresponding setter method of a filter.
The plugins JAlbum directory contains not only the filter classes but also the source code for the filters described on this page. Feel free to use them as base for your own filters. You will probably find the SimpleFilter below a good base for your own filters. The plugins folder also contains a compile.bat file that simplifies compilation for you. As you develop filters, please note that you do not need to restart JAlbum in order to refresh the filter classes as they are dynamically loaded every time an album is being processed. Finally, if you make a cool filter, please send it to me for inclusion.
Sample filter source code
The following simple filter adds a JAlbum logo to the corner of images:
import se.datadosen.jalbum.JAFilter;
import java.awt.*;
import java.awt.image.BufferedImage;
import javax.swing.ImageIcon;
/**
* JAlbum simple image filter adding a JAlbum logo to the
* corner of images
* @author David Ekholm
* @version 1.0
*/
public class SimpleFilter implements JAFilter {
// Implements JAFilter
public String getName() {
return "Simple filter";
}
// Implements JAFilter
public String getDescription() {
return "Add a JAlbum logo to the corner of images";
}
// Implements JAFilter
public BufferedImage filter(BufferedImage bi, java.util.Map vars) {
Graphics2D g = bi.createGraphics();
ImageIcon windowIcon =
new ImageIcon(se.datadosen.jalbum.JAlbumFrame.class.getResource(
"smallicon.gif"));
g.drawImage(windowIcon.getImage(), 0, 0, null);
return bi;
}
}
|